Progressive Submission
This tutorial explains how to use the progressive submission endpoints. These endpoints are available as part of the Conversations API.
Only API keys on our Conversations platform are eligible to use this API version. Refer to the Platforms section of our Platform & API Concepts documentation to learn which platform your API keys are on.
Overview
Progressive submission improves review content collection in two ways:
- By allowing collection of reviews on multiple products; and
- By allowing incremental submission of review attributes
The combination of these two functionalities makes it especially valuable for clients that sell multiple items to a consumer at once. This will improve review coverage across products as well as the content submission rate.
Additionally, the incremental submission functionality may be used to enhance existing single product review submissions. This will improve content submission using this simplified approach.
The flow-chart below is a summary of a multi-product submission process using the progressive submission endpoints:
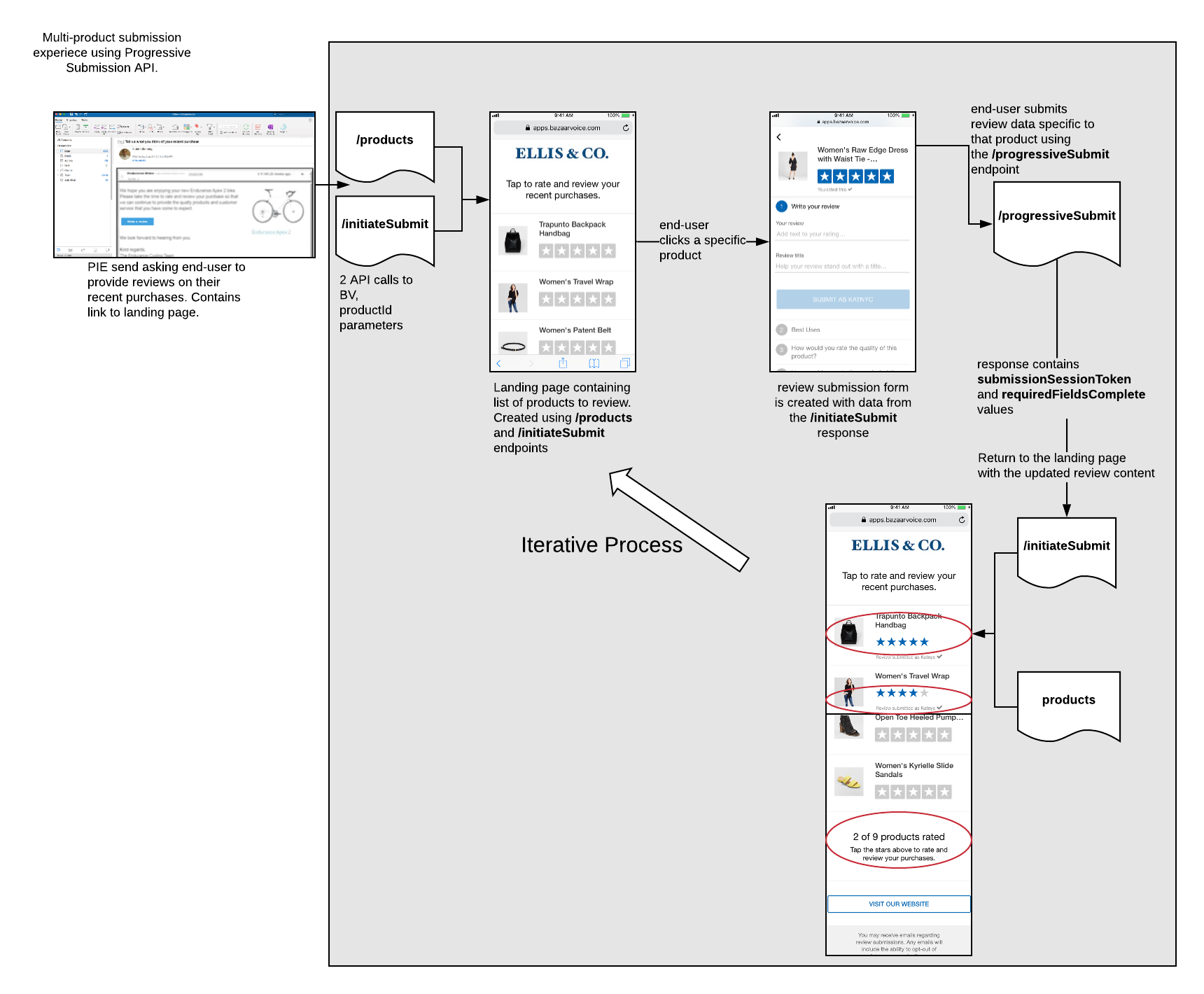
As previously described, progressive submission also supports the ability to collect review partials (i.e. ratings, review text, title, context data values) over multiple sessions. Reviews can be submitted for moderation once the required fields are completed. This is determined by the isFormComplete
field. Partial reviews can be iterated over using the submissionSessionToken
.
Components
The following table summarizes the components you will need to understand in order to implement progressive submission. These components will be referenced throughout the rest of this documentation.
Component | Description | Responsibility |
---|---|---|
Submission form | This a web form you build and host. Authors will use it to submit reviews. | client |
UserToken | The UserToken is an encoded set of fields sent in the body that are authenticated via an HMAC generated with the client's encodingKey. Must contain userId. Read more about userToken.The userToken and userId fields are mutually exclusive. Use one or the other. Using both will result in an error. | client |
UserId | Unique id for user. Can either be sent seprately at this level, or be encoded in UserToken. The userToken and userId fields are mutually exclusive. Use one or the other. Using both will result in an error. | client |
submissionSessionToken | Unique token ID required for progressive submission of the review. | Bazaarvoice/client |
preview parameter | Query string parameter indicating if content should be submitted for moderation. Used with the progressiveSubmit.json endpoint. Read more about the preview parameter. | Bazaarvoice/client |
isFormComplete parameter | A boolean value returned as a query string parameter indicating if content should be submitted for moderation. Used with the progressiveSubmit.json endpoint. | Bazaarvoice/client |
Walk-through
How it works
- Request reviews
Solicit reviews from consumers. - Initiate progressive submission
Request form data on product(s) using the initiateSubmit.json endpoint. For additional metadata about the product, you will also need to request data from the products endpoint. - Build product(s) submission form
Build the submission form(s) for the given productId(s). Render the HTML controls and values for the product submission form. - Submit product(s) review
Handle the productId(s) submissions. Use thepreview
parameter in the request to stage the review submission and theisFormComplete
in the response to observe if all the required fields are provided.
Step 1: Request reviews
Consumers are asked to provide reviews. This often comes through a post interactive email (PIE) containing a link to 'write reviews on your purchase'.
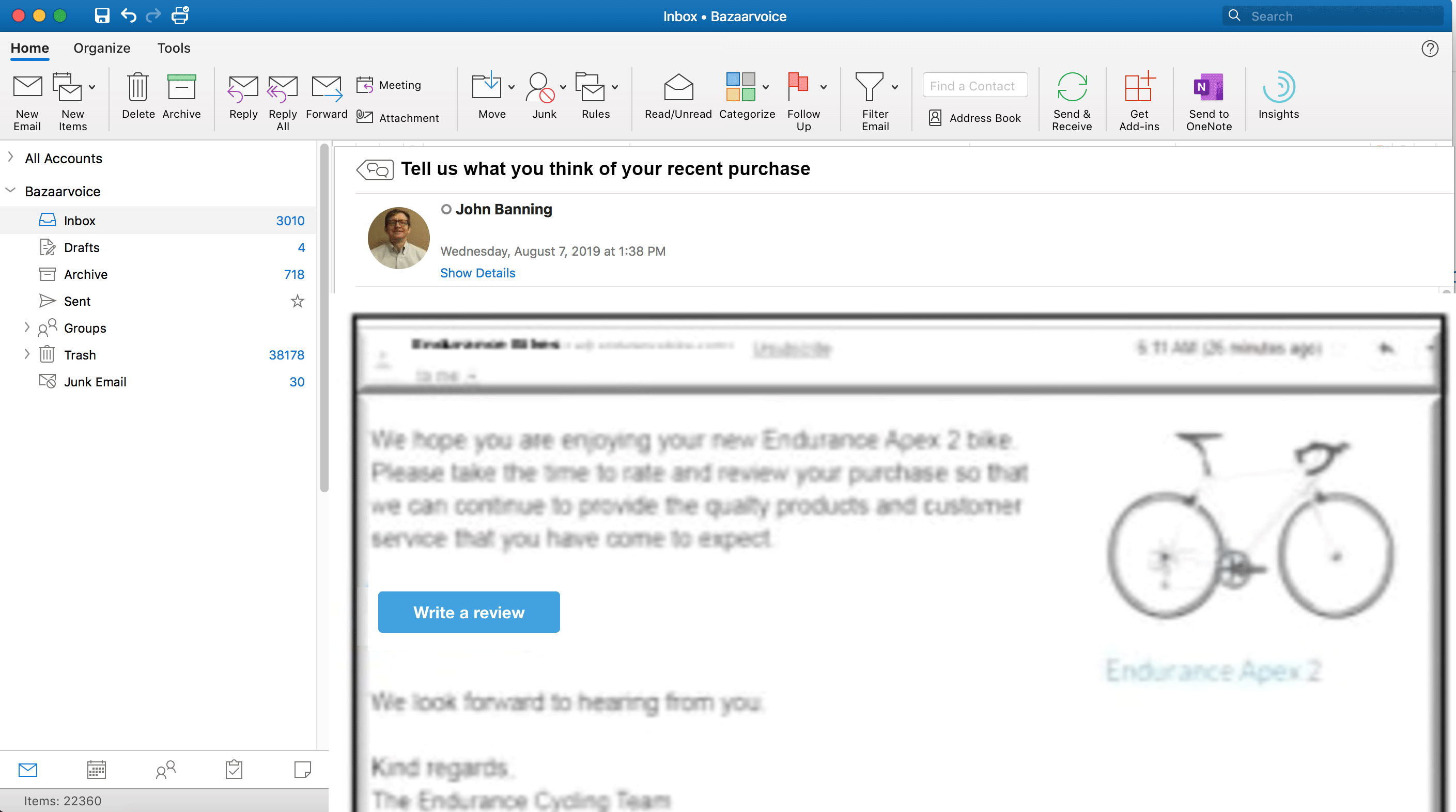
The user clicks the link in the email and is shown review submission forms for any number of products.
Other methods to solicit a shopper's feedback are also possible, such as hosting a recently purchased page where shoppers can leave reviews.
Step 2: Initiate Progressive Submission
Products may have different review attributes. For instance while a review form for an article of clothing may ask 'how was the fit', that question does not make sense for an electronics good.
The purpose of the initiateSubmit.json call is to provide API users with data to create the review submission form for a given productId. The endpoint accepts an array of productIds in the body parameter.
Step 3: Build product(s) submission form
Building a progressive submission form is similar to building a submission form using the full submission endpoint. High level steps include:
- Obtaining product metadata from the products endpoint. This may include product descriptions, images and any other relevant data to help users write a review. Rather than displaying just the questions to review a product, it is often helpful to provide context. Product images, title, and price are just some attributes that help when writing a review.
Review form without any product context. Review form displaying product context (images, title).
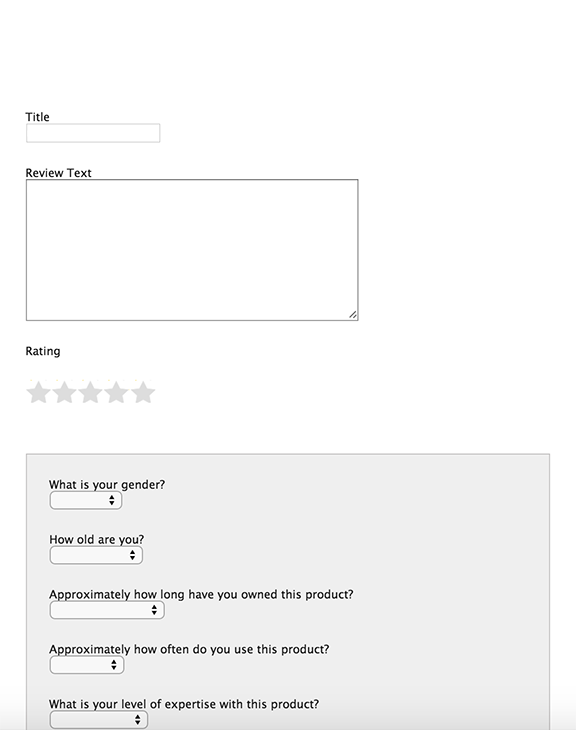
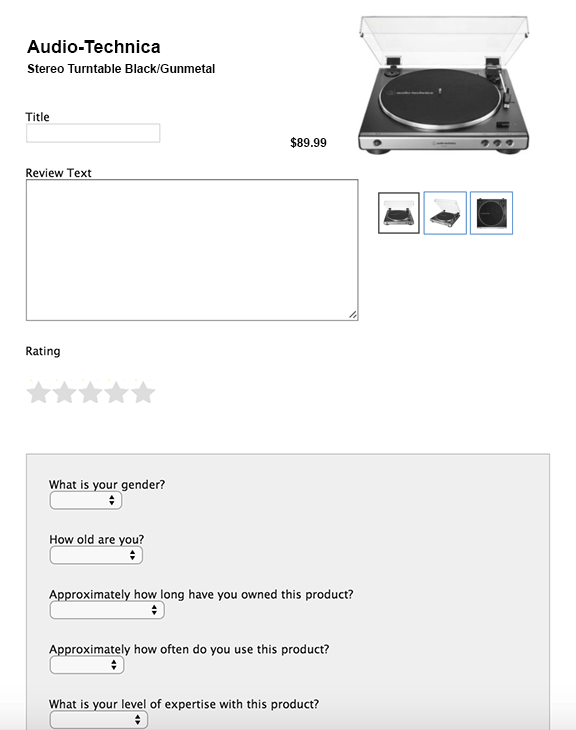
The products endpoint supports passing in a list of productIds to optimize the number of API requests.
- Use both the
fieldsOrder
andfields
elements from the initiateSubmit.json response to create the HTML controls needed for the submission form.
The fieldsOrder lists the required submission questions first, followed by those submission elements set up on the Site Manager.
The field data contains attributes that provide information on the specific HTML controls that should be displayed on the submission form.
The possible field types are shown below
"title": {
"required": true,
"id": "title",
"maxLength": 50,
"type": "text",
"class": "title"
},
As you can see, there is a list of name/value pairs that are returned for each Fields
object. The sole purpose of this data is to provide developers a blue print to construct a submission form.
Let's take a look at these name/value pairs:
hidden
The boolean value hidden
, indicates if the element should be displayed on the form. Only form elements with a true value are returned in the response. When not found in the response, hidden
is false.
"OrderNumber": {
"hidden": true,
"autoPopulate": false,
"answerLength": "SHORT_LINE",
"label": "Order Number",
"id": "additionalfield_OrderNumber",
"type": "text",
"class": "additionalField",
"required": false,
"maxLength": 35
},
autoPopulate
An autoPopulate
value makes it possible to pre-populated a control when building the form. An example of this is signing a user up to receive a newsletter.
newslettersignup: {
autoPopulate: true,
maxLength: null,
value: "true",
required: true,
type: "boolean",
label: null,
id: "newslettersignup",
minLength: null
},
value
The value
represents the user's answer to a question and is what Bazaarvoice will store. When obtaining pre-populated data such as making a request using the &User and/or &UserID parameter, the value is returned in the Value
key.
maxLength
The maxLength
is configured on the BV side and submissions will not be allowed unless it is met. The value can be used to create client side validation to ensure that the submission form meets the requirement. In the sample below the Review Text must not exceed 5000 characters.
reviewtext: {
default: null,
value: "",
maxLength: 50000,
required: false,
type: "TextAreaInput",
label: null,
id: "reviewtext",
minLength: 50
},
ERROR_FORM_TOO_LONG
error will be returned from the API if a submission exceeds themaxLength
value.
required
required
indicates that some value must be provided with submission of the form. Combination of required
and type
can be used to create client side validation.
commenttext: {
default: null,
value: null,
maxLength: 10000,
required: true,
type: "TextAreaInput",
label: null,
id: "commenttext",
minLength: 1
},
ERROR_FORM_REQUIRED
will be returned from the API if a submission is missing aRequired
field.
type
The type
name/value gives the developer an indication of the HTML control that should be used to capture the input on the form. These values can also be used to generate client side validation rules. Values returned within type
include:
* text
* choice
* boolean
* integer
* url
The Inputs Types tutorial is the authoritative source documenting the different field types.
label
The label
name/value will return labels for Additional fields, context data, tag dimensions and rating dimensions. If the label is "null", it is up to developers to provide values.
id
The id
value can be used to uniquely identify any controls used to build the form. This is also the value developers would use as the key in the submission (i.e id=value). In the HTML it would be the value of the name attribute.
valuesLabels
The valuesLabels
is an array of values that have been configured for the parent field
element. An example when to use the valuesLabels
object, is in forms that contains a drop-down list control. Programmatically a developer would iterate over the array extracting out the values and label to construct HTML. An example is shown below:
{
"valuesLabels": {
"1week": "1 week",
"1month": "1 month",
"3months": "3 months",
"6months": "6 months",
"1year": "1 year or longer"
},
"autoPopulate": false,
"label": "Approximately how long have you owned this product?",
"id": "contextdatavalue_LengthOfOwnership",
"type": "choice",
"class": "cdv",
"required": false
},
So programmatically you could end up with something like:
How old are you?
Please Select
1 week
1 month
3 month
6 months
1 year or longer
"choice" is the
type
value when thevaluesLabels
array is populated.
minLength
The minLength
is configured on the Bavaarvoice side and submissions will not be allowed unless it is met. The value can be used to create client-side validation to ensure that the submission form meets the requirements as defined in the configuration. It's preferred that form contain as much validation as possible before submitting. In the sample below the Review Text must be at least 50 characters.
reviewtext: {
default: null,
value: "",
maxLength: 50000,
required: false,
yype: "TextAreaInput",
label: null,
id: "reviewtext",
minLength: 50
},
ERROR_FORM_TOO_SHORT
error will be returned from the API if a submission does not meet theMinLength
value.
Step 4: Submit product(s) review
By this time, the review submission form(s) should be presented to the consumer to fill out. Again, this may be a single review form or a collection of submission forms.
The significance of progressive submission is that it allows shoppers the ability to partially submit a review and then return to that review to update it up to 30 days after the initial submission. For instance, it is possible to submit a review where all the required fields are not completed, then return to that review and complete the review.
The following discussion assumes the progressive submission described below:
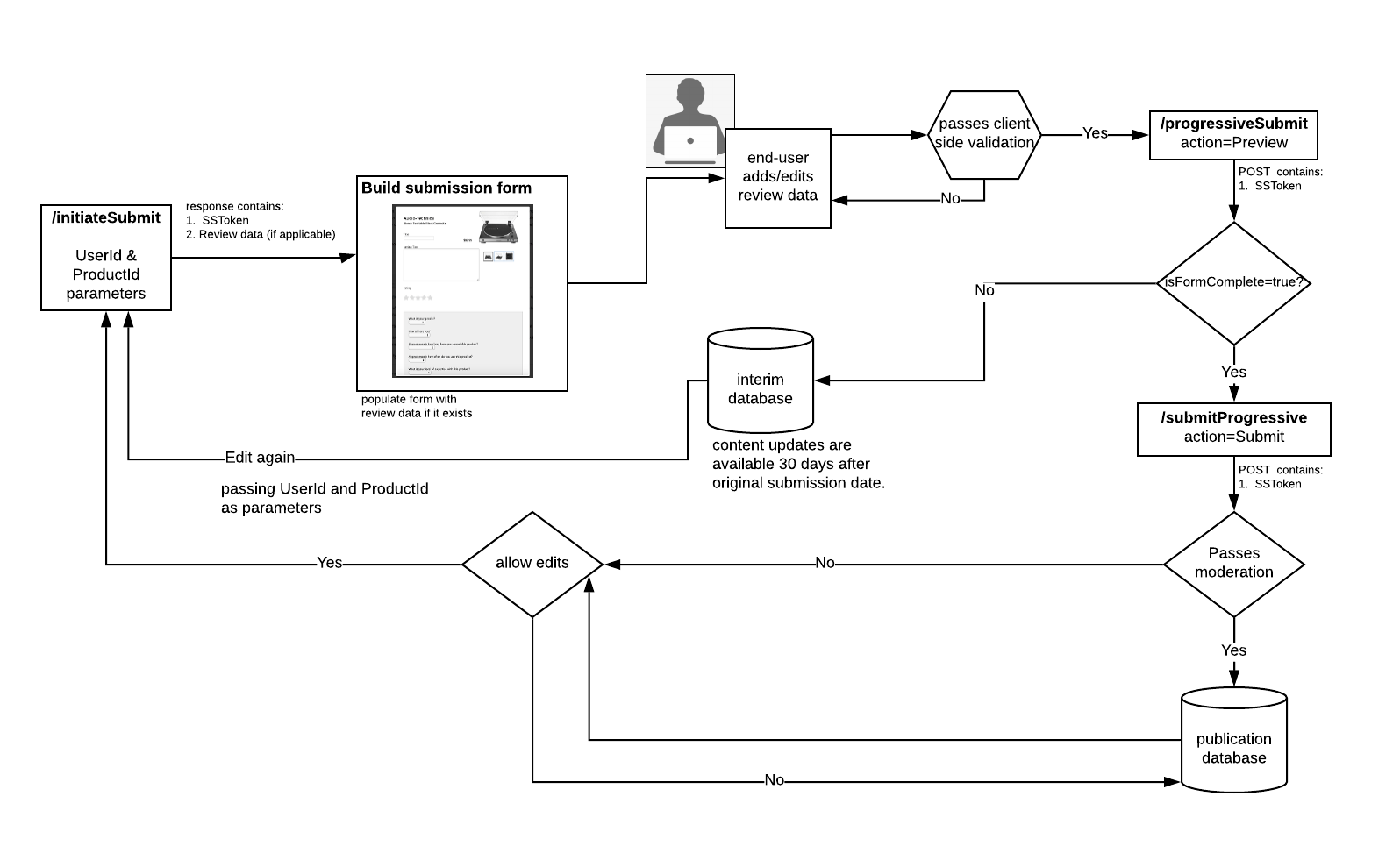
Product review submission forms are unique and require client-side validation that must be handled by clients.
Product review submission can be done using either BV Mastered(Hosted) Authentication and Client-Mastered Authentication.
The following reference documentation provides quick access to product review submission usage information either using BV Mastered(Hosted) Authentication OR Client-Mastered Authentication.
BV-Mastered Authentication This is useful for sites whose logins are difficult to customize or for sites that don't have account creation capabilities. | Client Mastered Authentication In this authentication type, user IDs come from your authentication system, which is considered to be the authoritative (master) source for user identification. |
---|
Updated 3 months ago