CORS
This tutorial explains how developers can use Cross Origin Resource Sharing (CORS) to make cross-domain GET and POST requests to the Conversations API.
Overview
CORS is a standardized way for a server to tell a browser to relax the Same Origin Policy.
There are two types of CORS:
- "Simple" CORS for requests similar to the GET and POST requests that would be performed by an HTML form tag.
- "Preflighted" CORS for requests that include custom, forbidden, or unsafe headers, special content types, or HTTP methods other than GET and POST. These types of requests are referred to as "preflighted" because the browser performs an initial OPTIONS request to coordinate the cross-domain communication with the server prior to performing the request initiated by your application.
In either type, the browser includes CORS specific headers with the request to initiate CORS and the server responds with CORS specific headers which provide the browser with instructions on if and how to relax the Same Origin Policy.
For more information about CORS refer to
https://developer.mozilla.org/en-US/docs/Web/HTTP/Access_control_CORS
CORS with the Conversations API
Web-browsers will automatically initiate CORS for cross domain requests to the Conversations API when they are performed with JavaScript. To avoid unnecessary OPTIONS requests and other errors, you should construct your API requests so they are compatible with the requirements for "simple" CORS, including:
- Use the HTTP GET or POST methods
- Do not use custom, forbidden, or unsafe headers
- With POST, use the
application/x-www-form-urlencoded
ormultipart/form-data
content types
A complete list of the requirements for "simple" CORS requests can be found at https://developer.mozilla.org/en-US/docs/Web/HTTP/Access_control_CORS#Simple_requests and some common issues are listed below.
In some cases it may be possible to perform API requests that trigger successful "preflighted" CORS OPTIONS requests. However, doing so is not necessary and will result in extra OPTIONS requests that reduce your perceived response time and will count against your API rate limit.
Identifying CORS issues
CORS related issues will typically demonstrate some or all of the following:
- Reference to headers that start with "Access-Control-"
- An OPTIONS request
The following screenshots show CORS related errors in the consoles of several popular web browsers.

CHROME
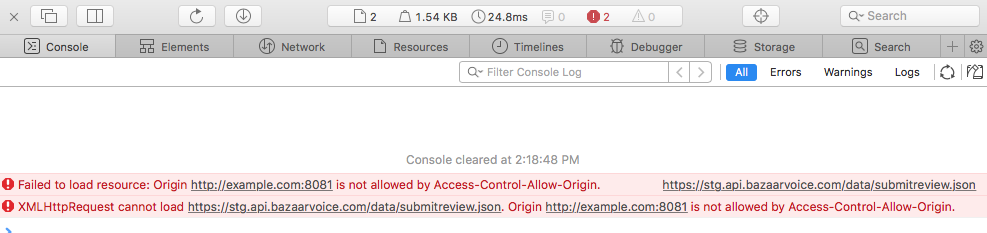
SAFARI

FIREFOX

EDGE
Common Issues
The following issues will trigger a "preflighted" CORS request which is unnecessary and may result in errors depending on the format of your request.
Using custom, forbidden, or unsafe headers
Any custom, forbidden, or unsafe headers will trigger an unnecessary preflight OPTIONS request and an error. A complete list of forbidden, or unsafe headers can be found at https://fetch.spec.whatwg.org/#cors-safelisted-request-header.
The following custom headers in particular are a common cause of issues:
X-Requested-With
The X-Requested-With
header is used to communicate the technology that initiated the request. This technique should not be used with the Conversations API because it will trigger an unnecessary preflight OPTIONS request and an error.
X-Forwarded-For
The X-Forwarded-For
header is used to communicate the IP address of the device originating an HTTP request. Bazaarvoice asks clients to include this header with the end-user's IP address when performing a server to server submission, because otherwise we would register all submissions as coming from the server's IP address.
However, when using CORS, the submission comes from the end-user's browser, so we automatically get the end-user's IP address. When using CORS
X-Forwarded-For
is unnecessary.
Using HTTP methods other than GET or POST
The Conversations API supports HTTP GET and POST. Using a method other than GET or POST will trigger an unnecessary preflight options request which may result in unexpected errors.
Incorrect Content-Type header value
When performing a submission, you should only use the following values with the Content-Type header in the indicated scenarios:
Value | Scenario |
---|---|
application/x-www-form-urlencoded | Use this value when submitting consumer generated content, such as Reviews, Questions, Comments and Answers. ![]() |
multipart/form-data | Use this value when uploading photo data. |
Any other Content-Type header value will trigger an unnecessary OPTIONS request.
Updated 3 months ago